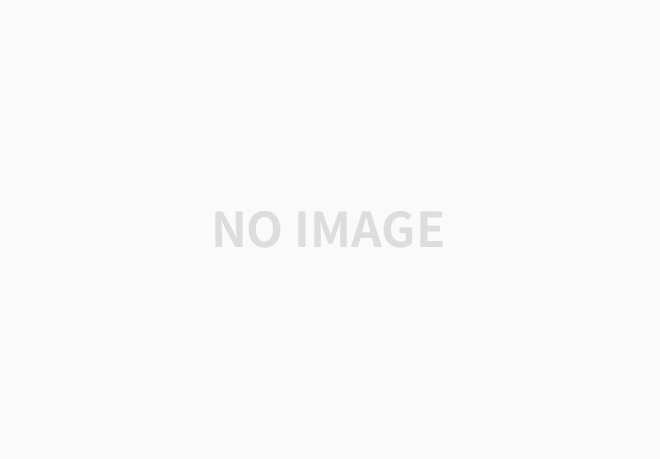
Dart는 모바일 애플리케이션 개발로 많이 알려져 있지만, 서버 사이드 개발에도 유용합니다.
Dart의 서버 프레임워크를 사용하면 서버 애플리케이션을 구조화된 방식으로 개발할 수 있습니다.
이번 포스트에서는 Aqueduct와 Shelf와 같은 Dart 서버 프레임워크를 이용하여 구조화된 서버를 개발하는 방법을 초보자도 이해할 수 있도록 설명하겠습니다.
서버 애플리케이션 구조화란?
서버 애플리케이션 구조화는 애플리케이션을 효율적으로 관리하고 유지보수하기 위해 코드와 기능을 체계적으로 나누는 과정을 의미합니다. 구조화된 서버 애플리케이션은 다음과 같은 이점을 제공합니다:
- 유지보수 용이성: 코드가 잘 조직되어 있어 수정이나 확장이 용이합니다.
- 확장성: 애플리케이션의 기능을 쉽게 추가하거나 변경할 수 있습니다.
- 재사용성: 공통 기능을 모듈화하여 여러 곳에서 재사용할 수 있습니다.
Aqueduct를 이용한 구조화된 서버 개발
Aqueduct는 강력한 기능을 갖춘 Dart 서버 프레임워크로, 구조화된 서버 애플리케이션을 개발하는 데 유용합니다.
Aqueduct를 사용하여 구조화된 서버를 개발하는 기본적인 방법을 살펴보겠습니다.
1. 프로젝트 구조 이해하기
Aqueduct 프로젝트는 일반적으로 다음과 같은 구조를 가집니다.
my_project/
├── lib/
│ ├── channel.dart
│ ├── controller/
│ ├── model/
│ └── ...
├── test/
├── pubspec.yaml
└── ...
- lib/channel.dart: 서버의 주요 진입점입니다. 여기서 요청 처리와 라우팅을 설정합니다.
- lib/controller/: 요청을 처리하는 컨트롤러들을 모아두는 폴더입니다.
- lib/model/: 데이터 모델을 정의하는 폴더입니다.
2. 컨트롤러와 모델 작성하기
Aqueduct에서 컨트롤러와 모델을 분리하여 작성함으로써 구조화된 애플리케이션을 만들 수 있습니다.
컨트롤러 작성하기
컨트롤러는 HTTP 요청을 처리하는 역할을 합니다. lib/controller/ 폴더에 새로운 컨트롤러 파일을 생성합니다.
예를 들어, lib/controller/hello_controller.dart 파일을 다음과 같이 작성합니다.
import 'package:aqueduct/aqueduct.dart';
class HelloController extends ResourceController {
@Operation.get()
Future<Response> getHello() async {
return Response.ok({'message': 'Hello, Aqueduct!'});
}
}
모델 작성하기
모델은 데이터베이스와 상호작용하는 데이터 구조를 정의합니다. lib/model/ 폴더에 새로운 모델 파일을 생성합니다.
예를 들어, lib/model/person.dart 파일을 다음과 같이 작성합니다.
import 'package:aqueduct/aqueduct.dart';
class Person extends ManagedObject<_Person> implements _Person {}
class _Person {
@primaryKey
int id;
@Column(unique: true)
String name;
}
3. 애플리케이션 설정하기
lib/channel.dart 파일에서 라우팅을 설정하고, 컨트롤러와 모델을 애플리케이션에 연결합니다.
import 'package:aqueduct/aqueduct.dart';
import 'controller/hello_controller.dart';
class MyChannel extends ApplicationChannel {
@override
Future prepare() async {}
@override
Controller get entryPoint {
final router = Router();
router.route('/hello').link(() => HelloController());
return router;
}
}
4. 애플리케이션 실행하기
터미널에서 다음 명령어를 입력하여 애플리케이션을 실행합니다.
aqueduct serve
이 명령어는 localhost:8888에서 서버를 실행합니다. 웹 브라우저에서 http://localhost:8888/hello에 접속하여 "Hello, Aqueduct!" 메시지를 확인할 수 있습니다.
Shelf를 이용한 구조화된 서버 개발
Shelf는 모듈화된 웹 서버를 구축할 수 있는 프레임워크입니다. Shelf를 사용하여 구조화된 서버를 개발하는 기본적인 방법을 살펴보겠습니다.
1. 프로젝트 구조 이해하기
Shelf 프로젝트는 일반적으로 다음과 같은 구조를 가집니다.
my_shelf_project/
├── bin/
│ └── server.dart
├── lib/
│ ├── controller/
│ └── ...
├── pubspec.yaml
└── ...
- bin/server.dart: 서버의 진입점입니다. 서버를 설정하고 실행합니다.
- lib/controller/: 요청을 처리하는 컨트롤러들을 모아두는 폴더입니다.
2. 컨트롤러 작성하기
Shelf에서는 컨트롤러를 직접 작성하지 않고, 미들웨어와 핸들러를 사용하여 요청을 처리합니다. lib/controller/ 폴더에 새로운 핸들러 파일을 생성합니다.
예를 들어, lib/controller/hello_handler.dart 파일을 다음과 같이 작성합니다.
import 'package:shelf/shelf.dart';
Response helloHandler(Request request) {
return Response.ok('Hello, Shelf!');
}
3. 애플리케이션 설정하기
bin/server.dart 파일에서 미들웨어와 핸들러를 설정합니다.
import 'package:shelf/shelf.dart';
import 'package:shelf/shelf_io.dart' as io;
import 'package:shelf/shelf.dart' as shelf;
import 'package:my_shelf_project/controller/hello_handler.dart';
void main() async {
final handler = const Pipeline()
.addMiddleware(logRequests())
.addHandler(helloHandler);
final server = await io.serve(handler, 'localhost', 8080);
print('Server running on http://${server.address.host}:${server.port}');
}
4. 애플리케이션 실행하기
위의 코드를 작성한 후, 터미널에서 다음 명령어를 입력하여 애플리케이션을 실행합니다.
dart bin/server.dart
이 명령어는 localhost:8080에서 서버를 실행합니다.
웹 브라우저에서 http://localhost:8080에 접속하여 "Hello, Shelf!" 메시지를 확인할 수 있습니다.
Dart의 서버 프레임워크인 Aqueduct와 Shelf를 사용하면 구조화된 서버 애플리케이션을 쉽게 개발할 수 있습니다.
Aqueduct는 강력한 기능을 제공하며 복잡한 애플리케이션에 적합하고, Shelf는 간단하고 모듈화된 구조를 통해 기본적인 웹 서버를 신속하게 구축할 수 있습니다.
구조화된 서버 개발을 통해 코드의 유지보수성과 확장성을 높일 수 있습니다.
이 블로그 포스트가 Dart 서버 프레임워크를 이용한 구조화된 서버 개발에 대한 이해를 돕는 데 도움이 되길 바랍니다!
구독!! 공감과 댓글은 저에게 큰 힘이 됩니다.
Starting Google Play App Distribution! "Tester Share" for Recruiting 20 Testers for a Closed Test.
Tester Share [테스터쉐어] - Google Play 앱
Tester Share로 Google Play 앱 등록을 단순화하세요.
play.google.com
'Dart > Dart Server' 카테고리의 다른 글
[중급] Dart 서버 데이터베이스 연동/ ORM(Object-Relational Mapping) 도구 사용법 (Aqueduct ORM 등) (0) | 2024.09.11 |
---|---|
[중급] Dart 서버 데이터베이스 연동/ Dart와 관계형 데이터베이스 연결 (PostgreSQL, MySQL 등) (1) | 2024.09.11 |
[중급] Dart 서버 프레임워크 이해하기/ 각 프레임워크의 설치 및 기본 사용법 (0) | 2024.09.09 |
[중급] Dart 서버 프레임워크 이해하기/대표적인 Dart 서버 프레임워크 소개 (Aqueduct, Shelf ) (2) | 2024.09.09 |
[초급] Dart RESTful API 설계 기초/ 경로 매개변수 및 쿼리 매개변수 처리 (0) | 2024.09.08 |